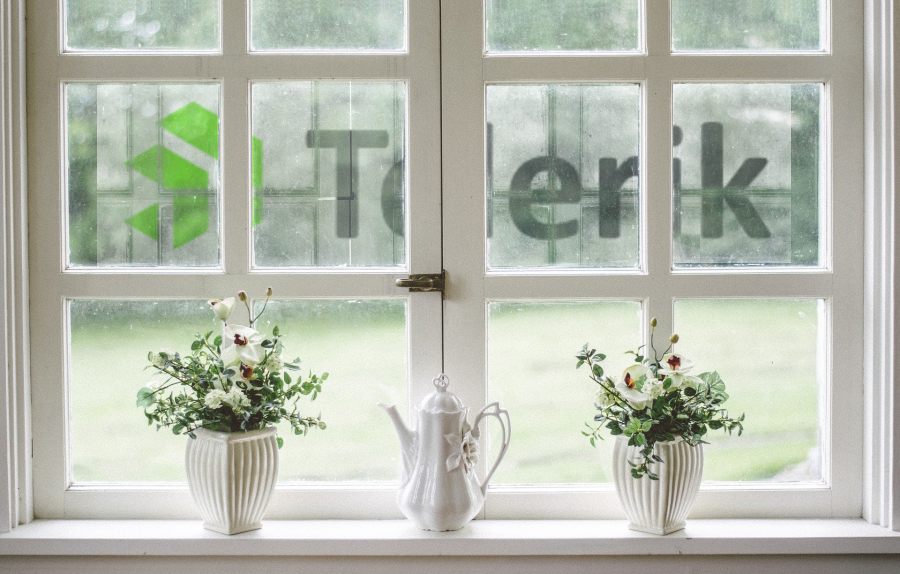
Showing the progress of your application gives the user a better experience. They can continue with other tasks and still see where your app is up to.
Adding this for a regular WPF Window is quite simple, you can create a TaskbarItemInfo in markup:
<Window.TaskbarItemInfo>
<TaskbarItemInfo x:Name="taskBarItemInfo" />
</Window.TaskbarItemInfo>
The ProgressValue takes a double between 0 – 1 where 0 has no green bar and 1 is a full bar.
The ProgressState takes an enum
of TaskbarItemProgressState which can be:
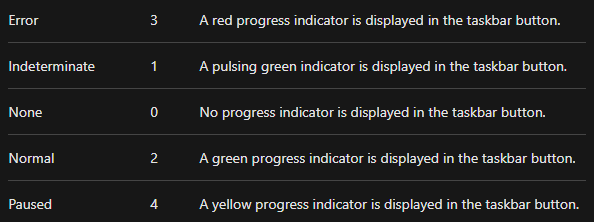
So setting the ProgressState to normal
, and the ProgressValue to .50
taskbarItemInfo.ProgressState = TaskbarItemProgressState.Normal;
taskbarItemInfo.ProgressValue = .5;
Will look like this:

We can then set the ProgressValue to update according to the task we’re processing and set the ProgressState back to none
when done.
The RadWindow
though does not expose a TaskbarItemInfo property. It is primarily a child window, and whilst it can used as a main Window it does not have all the properties a regular WPF Window has.
To get around this issue we need to tap into the parent of the RadWindow
, this is a regular WPF Window. Then we can set the TaskbarItemInfo up on that.
private void ShowProgressInTaskbar() {
Dispatcher.Invoke(() => {
Window window = this.ParentOfType<Window>();
if (window != null) {
window.TaskbarItemInfo = new() {
ProgressState = TaskbarItemProgressState.Normal,
ProgressValue = .5
};
}
});
}
We need to invoke the dispatcher since the code is running on a different thread than the UI. If your setting the ProgressValue elsewhere then that will also need to be wrapped in a Dispatcher.Invoke
:
while ((read = input.Read(buffer, 0, buffer.Length)) > 0) {
output.Write(buffer, 0, read);
totalRead += read;
int megabytesRead = totalRead / 1024 / 1024;
ProgressValue = megabytesRead * 100 / megabytesTotal;
BusyContent = title + Environment.NewLine + $"Saving {megabytesRead} MB / {megabytesTotal} MB ...";
Dispatcher.Invoke(() => {
Window.TaskbarItemInfo.ProgressValue = Math.Round(ProgressValue / 100d, 2);
});
}