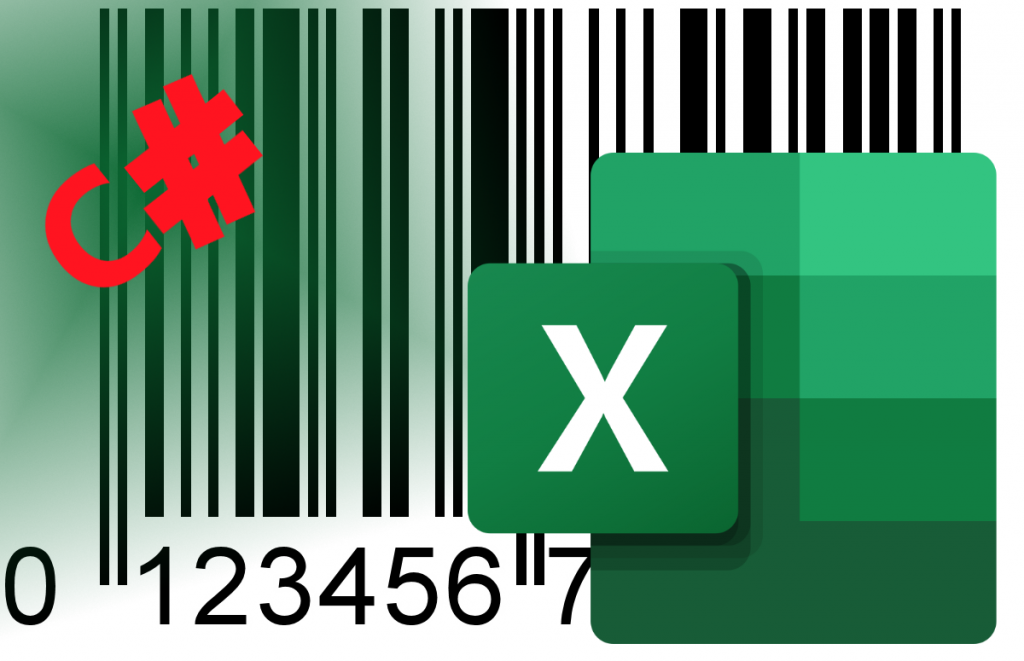
I was recently asked to build an app that can extract the data from a barcode image and save it to an Excel sheet. It turned out to be pretty simple. Having come across this tutorial, I adapted it slightly to fit my requirements.
First we need to install two packages with NuGet.
- FreeSpire.Barcode
- FreeSpire.XLS
I’m using WPF with .NET Core, but obviously the UI can be written with whatever you prefer.
Lets start by taking a look at the method:
private async Task Run() {
busyIndicator.IsBusy = true;
bool success = false;
Exception exception = new Exception();
await Task.Run(() => {
string[] picFile = Directory.GetFiles(BarcodeImageLoc);
List<string> list = new List<string>();
try {
for (int i = 0; i < picFile.Length; i++) {
string scanResult = BarcodeScanner.ScanOne(picFile[i]);
list.Add(scanResult);
picFile[i] = System.IO.Path.GetFileName(picFile[i]);
}
success = true;
} catch (Exception ex) {
success = false;
exception = ex;
}
if (success) {
string[] barcodeData = list.ToArray();
Workbook wb = new Workbook();
Worksheet sheet = wb.Worksheets[0];
sheet.Range["A1"].Text = "Name";
sheet.Range["B1"].Text = "Data";
sheet.Range["A1"].Style.Font.IsBold = true;
sheet.Range["B1"].Style.Font.IsBold = true;
sheet.InsertArray(picFile, 2, 1, true);
sheet.InsertArray(barcodeData, 2, 2, true);
sheet.Columns[0].ColumnWidth = 15f;
sheet.Columns[1].ColumnWidth = 20f;
sheet.Columns[1].IgnoreErrorOptions = IgnoreErrorType.NumberAsText;
string fullPath = SpreadsheetNameLoc;
wb.SaveToFile(fullPath, FileFormat.Version2010);
}
});
if (success) {
Alert(new DialogParameters {
Header = "Success",
Content = "Saving complete",
Owner = this
});
} else {
Alert(new DialogParameters {
Header = "Error",
Content = exception.Message + Environment.NewLine + "Please select a valid directory with valid barcode images and try again.",
Owner = this
});
}
busyIndicator.IsBusy = false;
}
We start off by setting the spinner to show the user we are processing the request. Then we set up a bool success = false
which we’ll use to check whether we have completed successfully or are there any errors, and an Exception
to store the errors.
Then we load the images into memory, and loop over them [line: 12] to obtain the file names extensions and the scan result (using the Spire.Barcode package we installed earlier) of the files stores in the picFile
array.
At this point any errors should have been caught and saved to the Exception
we created. If there are errors we skip the rest and show an alert with the errors.
If we succeeded, we need to convert the List
to an array
[line: 24].
Now we can start writing the array to Excel.
Create a new Workbook
(using the Spire.XLS package), and add column names in cells A1 and B1.
Invoke InsertArray(string[] stringArray, int firstRow, int firstColumn, bool isVertical) from Spire.XLS namespace to import the arrays of the strings to worksheet.
Finally save the workbook, and show an alert that process has completed successfully. Then stop the spinner.
At this point it would be nice to open the Excel sheet straight away to see the data.
System.Diagnostics.Process.Start(fullPath);
Adding this code though, only throws an exception. So for now I’ve left it out.