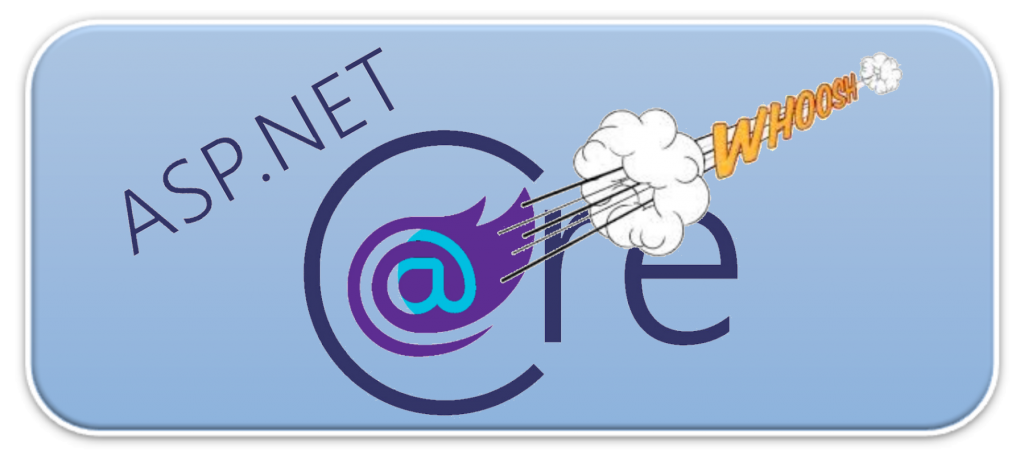
Creating the ability to add Blazor components to your existing app [thereby enabling you to work more efficiently – no Java Script!] takes no more than 5 minutes and a few lines of code!
First add the Blazor js file to the main _Layout.cshtml
file.
[From my experience this must be at the end of the file just before the closing <⁄body>
tag]
<script src="_framework/blazor.server.js"></script>
Next we need to add the services that Blazor require, in the Startup.cs
file
services.AddServerSideBlazor();
and add an endpoint for the SignalR connection:
endpoints.MapBlazorHub();
Add an _Imports.razor
file to the root of the project
@using System.Net.Http
@using Microsoft.AspNetCore.Components.Forms
@using Microsoft.AspNetCore.Components.Routing
@using Microsoft.JSInterop
@using Microsoft.AspNetCore.Components.Web
That is all that is needed to get setup. Now we can start adding Blazor components. Add a component called Counter.razor
and add the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | < h1 >Counter</ h1 > < p >Current count: @currentCount</ p > < button class = "btn btn-primary" @ onclick = "IncrementCount" >Click me</ button > @code { private int currentCount = 0; private void IncrementCount() { currentCount++; } } |
To use this component in the Index.cshtml
page add
<component type="typeof(Counter)" render-mode="ServerPrerendered" />
Run the app now and the Blazor component is displayed in the index page.
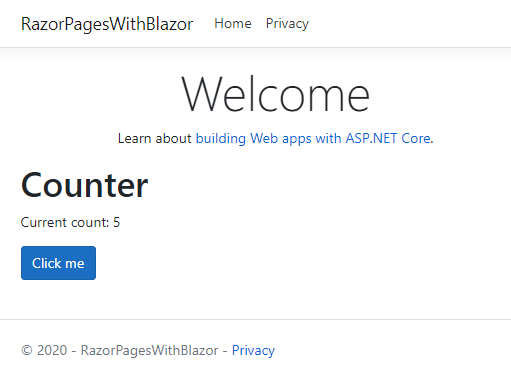